Hey, I'm new to the java programming language and am trying to create a frame that takes input and uses it as the radius to calculate the diameter and circumference of a circle. I get this compiler error:
CircleFrame.java:34: <identifier> expected
output.add(out1);
^
CircleFrame.java:34: <identifier> expected
output.add(out1);
^
CircleFrame.java:35: <identifier> expected
output.add(out2);
^
CircleFrame.java:35: <identifier> expected
output.add(out2);
^
CircleFrame.java:39: class, interface, or enum expected
public static void main(String[] arguments) {
^
CircleFrame.java:41: class, interface, or enum expected
}
^
6 errors
And this is my code:
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class CircleFrame extends JFrame implements ActionListener,
KeyListener {
public CircleFrame() {
super("Circle Calculator");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JTextField radius = new JTextField(
"Radius", 12);
radius.addKeyListener(this);
JPanel panel = new JPanel();
panel.add(radius);
add(panel);
pack();
setVisible(true);
}
public void keyReleased(KeyEvent keyPressed) {
Object source = event.getSource();
if (source instanceof JTextField) {
double inputRadius = input.getText();
output1 = inputRadius * 2;
output2 = output1 * 3.14;
break;
}
}
JMenu output = newJMenu("Output");
JMenuItem out1 = new JMenuItem(output1 + " is the radius");
JMenuItem out2 = new JMenuItem(output2 + " is the circumference");
output.add(out1);
output.add(out2);
}
public static void main(String[] arguments) {
CircleFrame frame = new CircleFrame();
}
}
I hope someone can help me!
CircleFrame.java:34: <identifier> expected
output.add(out1);
^
CircleFrame.java:34: <identifier> expected
output.add(out1);
^
CircleFrame.java:35: <identifier> expected
output.add(out2);
^
CircleFrame.java:35: <identifier> expected
output.add(out2);
^
CircleFrame.java:39: class, interface, or enum expected
public static void main(String[] arguments) {
^
CircleFrame.java:41: class, interface, or enum expected
}
^
6 errors
And this is my code:
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class CircleFrame extends JFrame implements ActionListener,
KeyListener {
public CircleFrame() {
super("Circle Calculator");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JTextField radius = new JTextField(
"Radius", 12);
radius.addKeyListener(this);
JPanel panel = new JPanel();
panel.add(radius);
add(panel);
pack();
setVisible(true);
}
public void keyReleased(KeyEvent keyPressed) {
Object source = event.getSource();
if (source instanceof JTextField) {
double inputRadius = input.getText();
output1 = inputRadius * 2;
output2 = output1 * 3.14;
break;
}
}
JMenu output = newJMenu("Output");
JMenuItem out1 = new JMenuItem(output1 + " is the radius");
JMenuItem out2 = new JMenuItem(output2 + " is the circumference");
output.add(out1);
output.add(out2);
}
public static void main(String[] arguments) {
CircleFrame frame = new CircleFrame();
}
}
I hope someone can help me!
Create an account or sign in to comment.
35
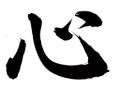
It was difficult to understand (not all the code was written out which made it difficult for me to read), and I just found some help on the Minecraft Forums, but thanks anyway!
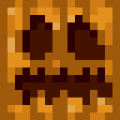
The point was that you'd take it and figure out what you needed to add, because I fixed the output bug.
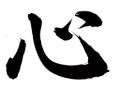
I've um... yeah. I'll find a tutorial
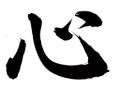
Thanks... but this isn't really my style.
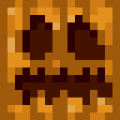
Not your style? It's practically identical to the type of code you had bar the actionlistener
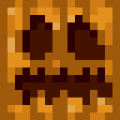
Here's a little program I wrote that takes in user input and makes it a label text. You should be able to use it to calculate what you need:
Click to reveal
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/* FrameDemo.java requires no other files. */
public class CircleFrame {
static String answer = "Text appears here";
/**
* Create the GUI and show it. For thread safety,
* this method should be invoked from the
* event-dispatching thread.
*/
private static void createAndShowGUI() {
//Create and set up the window.
JFrame frame = new JFrame("FrameDemo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
final JLabel emptyLabel = new JLabel(answer);
emptyLabel.setPreferredSize(new Dimension(175, 100));
frame.getContentPane().add(emptyLabel, BorderLayout.CENTER);
final JTextField field = new JTextField();
field.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
emptyLabel.setText(field.getText());
}
});
frame.add(field, BorderLayout.SOUTH);
//Display the window.
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
//Schedule a job for the event-dispatching thread:
//creating and showing this application's GUI.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}
import java.awt.event.*;
import javax.swing.*;
/* FrameDemo.java requires no other files. */
public class CircleFrame {
static String answer = "Text appears here";
/**
* Create the GUI and show it. For thread safety,
* this method should be invoked from the
* event-dispatching thread.
*/
private static void createAndShowGUI() {
//Create and set up the window.
JFrame frame = new JFrame("FrameDemo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
final JLabel emptyLabel = new JLabel(answer);
emptyLabel.setPreferredSize(new Dimension(175, 100));
frame.getContentPane().add(emptyLabel, BorderLayout.CENTER);
final JTextField field = new JTextField();
field.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
emptyLabel.setText(field.getText());
}
});
frame.add(field, BorderLayout.SOUTH);
//Display the window.
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
//Schedule a job for the event-dispatching thread:
//creating and showing this application's GUI.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}
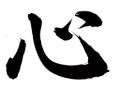
so, how'd it turn out.
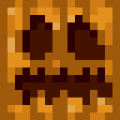
I can't see what the problem is at the moment so I'm writing the program again the way I'd do it to see if it works.
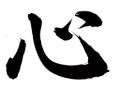
... well, I've not learned anything so far.
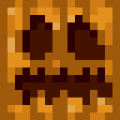
Then you should go and lookup some Java tutorials
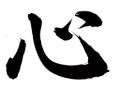
Here, how about I give the file, and someone returns a revised copy?
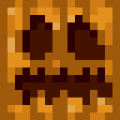
You won't learn anything if we just fix it for you.
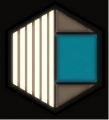
About one exactly.
Honestly though, can either of you
help me with JDK? It seems that
no matter how many times i link it
to the path it still can't find it. (on command prompt)
Honestly though, can either of you
help me with JDK? It seems that
no matter how many times i link it
to the path it still can't find it. (on command prompt)
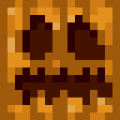
Did you link it to the bin folder followed by ; ?
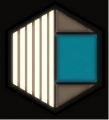
Has no one read at how bad I actually am.
Goddamn you lot, trying to help people
gives me a stick up my ass.
Goddamn you lot, trying to help people
gives me a stick up my ass.
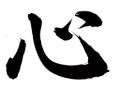
No more replies? I still need help.
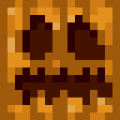
You have the Circle Frame class, and it has a main method in it. In the main method you run :
CircleFrame frame = new CircleFrame();
Which creates a new object of the class and runs the main method again
CircleFrame frame = new CircleFrame();
Which creates a new object of the class and runs the main method again
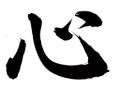
So what are you saying? Should I not have the main method in the class?
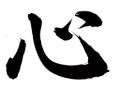
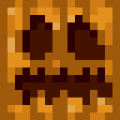
You're going to need to make a second class with the main method in it, you can't have it inside an object.
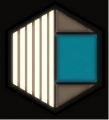
Honestly? I don't know. If you'd asked me last year maybe I would.
I can't even get JDK running on my computer anymore.
In any case, i remember i was using a language called
choicescript once and it was very *ahem* anal *ahem*
about spaces. Occasionally i got errors because of it.
Just wanted to share.
I can't even get JDK running on my computer anymore.
In any case, i remember i was using a language called
choicescript once and it was very *ahem* anal *ahem*
about spaces. Occasionally i got errors because of it.
Just wanted to share.
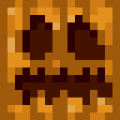
Well that's choicescript, not java
@Op I had a poke around in the source, there are a fair few bugs and while I've gotten it down to 2 errors it's going to get worse when I fix those. Have a look at some KeyListener tutorials and the likes
@Op I had a poke around in the source, there are a fair few bugs and while I've gotten it down to 2 errors it's going to get worse when I fix those. Have a look at some KeyListener tutorials and the likes
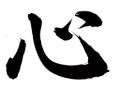
I have a class called Circle with a main method in it. Will that help?
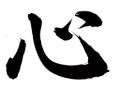
It's in an object? It doesn't appear so to me.

boy if java looked like PHP that would of been so much easier for everyone to code in it
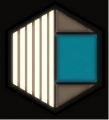
I know this may sound weird, but try not leaving a gap before the *
What the guy above said is true, but it could also be that it
cant read the imput
What the guy above said is true, but it could also be that it
cant read the imput
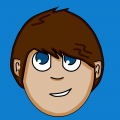
Leaving a space really doesn't change anything...
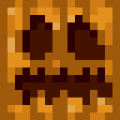
We worked that out a few post ago
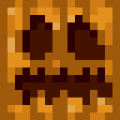
The gap between * doesn't matter.
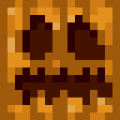
Post it on pastebin, but the error is because you don't have a variable or object called output.
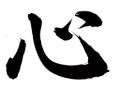
I don't understand what you mean and here:
pastebin.com/yjT8BnS0
pastebin.com/yjT8BnS0
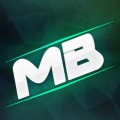
A lot of people don't know coding lol you'll have to go onto a Java Forums or some forums about coding
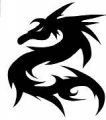
The source formatting is a pain to read this way. Try to post the source via pastebin.com and post the pastebin link here. Then more people might actually try to read it
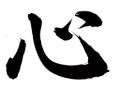
Why am I getting no replies...
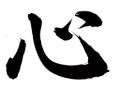
I fixed the double closing braces, and it still didn't work, so ignore the second one.